Python Try- Error Except Try Again
Summary: in this tutorial, you'll learn how to use the Python try...except
statement to handle exceptions gracefully.
In Python, at that place're 2 main kinds of errors: syntax errors and exceptions.
Syntax errors
When you write an invalid Python code, you'll go a syntax fault. For example:
current = one if current < x electric current += 1
Code language: Python ( python )
If you lot try to run this code, you lot'll get the following error:
Lawmaking linguistic communication: Trounce Session ( shell )
File "d:/python/effort-except.py", line 2 if electric current < 10 ^ SyntaxError: invalid syntax
In this example, the Python interpreter detected the mistake at the if
statement since a colon (:
) is missing after it.
The Python interpreter shows the file name and line number where the fault occurred and so that y'all can ready it.
Exceptions
Even though when your code has valid syntax, it may cause an fault during execution.
In Python, errors that occur during the execution are chosen exceptions. The causes of exceptions mainly come from the environment where the code executes. For instance:
- Reading a file that doesn't exist.
- Connecting to a remote server that is offline.
- Bad user inputs.
When an exception occurs, the program doesn't handle it automatically. This results in an mistake bulletin.
For example, the post-obit programme calculates the sales growth:
# get input cyberspace sales print('Enter the net sales for') previous = float(input('- Prior menses:')) electric current = float(input('- Current period:')) # calculate the alter in percentage change = (current - previous) * 100 / previous # bear witness the upshot if change > 0: result = f'Sales increase {abs(change)}%' else: outcome = f'Sales decrease {abs(change)}%' print(outcome)
Code language: Python ( python )
How information technology works.
- Beginning, prompt users for entering two numbers: the net sales of the prior and current periods.
- So, summate the sales growth in percentage and bear witness the event.
When you lot run the program and enter 120'
as the net sales of the current period, the Python interpreter will issue the post-obit output:
Code linguistic communication: Shell Session ( shell )
Enter the net sales for - Prior menses:100 - Current period:120' Traceback (most recent call concluding): File "d:/python/try-except.py", line 5, in <module> current = float(input('- Current menstruation:')) ValueError: could not convert string to bladder: "120'"
The Python interpreter showed a traceback that includes detailed data of the exception:
- The path to the source code file (
d:/python/try-except.py
) that caused the exception. - The verbal line of code that caused the exception (
line 5
) - The statement that caused the exception
current = float(input('- Current period:'))
- The type of exception
ValueError
- The mistake message:
ValueError: could not convert string to float: "120'"
Because float()
couldn't catechumen the string 120'
to a number, the Python interpreter issued a ValueError
exception.
In Python, exceptions take different types such every bit TypeError
, NameError
, etc.
Handling exceptions
To make the programme more than robust, you demand to handle the exception in one case it occurs. In other words, you need to catch the exception and inform users so that they tin fix it.
A skillful way to handle this is not to show what the Python interpreter returns. Instead, y'all replace that error message with a more user-friendly one.
To do that, you lot tin employ the Python try...except
argument:
try: # code that may cause mistake except: # handle errors
Code language: Python ( python )
The try...except
argument works equally follows:
- The statements in the
endeavor
clause executes offset. - If no exception occurs, the
except
clause is skipped and the execution of theeffort
statement is completed. - If an exception occurs at any statement in the
attempt
clause, the rest of the clause is skipped and theexcept
clause is executed.
The following flowchart illustrates the try...except
statement:
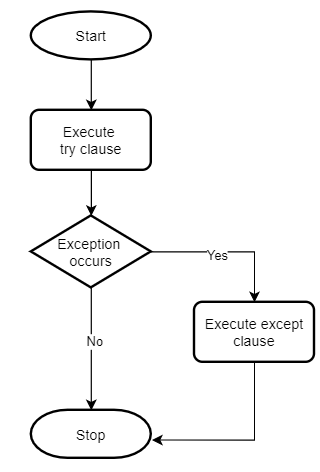
So to handle exceptions using the try...except
argument, you place the code that may crusade an exception in the effort
clause and the code that handles exception in the except
clause.
Hither's how y'all can rewrite the program and uses the try...except
statement to handle the exception:
try: # get input internet sales print('Enter the cyberspace sales for') previous = bladder(input('- Prior flow:')) current = float(input('- Electric current menstruation:')) # calculate the change in percentage alter = (current - previous) * 100 / previous # show the result if alter > 0: result = f'Sales increment {abs(modify)}%' else: issue = f'Sales decrease {abs(change)}%' impress(consequence) except: print('Fault! Please enter a number for net sales.')
Code language: Python ( python )
If you lot run the program once more and enter the net sales which is non a number, the program will outcome the bulletin that you specified in the except
cake instead:
Lawmaking language: Shell Session ( shell )
Enter the net sales for - Prior flow:100 - Electric current period:120' Mistake! Please enter a number for net sales.
Catching specific exceptions
When you enter the net sales of the prior period as nothing, you'll get the following message:
Code language: Vanquish Session ( crush )
Enter the cyberspace sales for - Prior menstruum:0 - Current period:100 Error! Please enter a number for cyberspace sales.
In this case, both net sales of the prior and current periods are numbers, the program still issues an error message. Another exception must occur.
The try...except
argument allows you to handle a detail exception. To catch a selected exception, you place the type of exception later the except
keyword:
try: # code that may crusade an exception except ValueError equally error: # code to handle the exception
Code language: Python ( python )
For instance:
try: # go input net sales print('Enter the net sales for') previous = bladder(input('- Prior catamenia:')) current = float(input('- Current period:')) # calculate the alter in percentage change = (current - previous) * 100 / previous # show the consequence if alter > 0: result = f'Sales increase {abs(change)}%' else: upshot = f'Sales decrease {abs(change)}%' print(result) except ValueError: print('Fault! Please enter a number for cyberspace sales.')
Code language: Python ( python )
When you run a programme and enter a string for the net sales, you'll go the same fault message.
However, if you enter cipher for the net sales of the prior catamenia:
Code language: Shell Session ( beat out )
Enter the net sales for - Prior flow:0 - Current menstruum:100
… you lot'll become the following error message:
Code language: Crush Session ( shell )
Traceback (most recent call concluding): File "d:/python/endeavor-except.py", line 9, in <module> alter = (current - previous) * 100 / previous ZeroDivisionError: float sectionalization by nil
This fourth dimension you got the ZeroDivisionError
exception. This division by zero exception is acquired by the post-obit statement:
alter = (current - previous) * 100 / previous
Lawmaking language: Python ( python )
And the reason is that the value of the previous
is zippo.
Treatment multiple exceptions
The attempt...except
allows yous to handle multiple exceptions by specifying multiple except
clauses:
try: # code that may cause an exception except Exception1 equally e1: # handle exception except Exception2 equally e2: # handle exception except Exception3 equally e3: # handle exception
Lawmaking language: Python ( python )
This allows you to answer to each type of exception differently.
If yous desire to have the same response to some type of exceptions, you can group them in one except clause:
try: # lawmaking that may cause an exception except (Exception1, Exception2): # handle exception
Lawmaking language: Python ( python )
The following example shows how to apply the try...except
to handle the ValueError
and ZeroDivisionError
exceptions:
try: # get input net sales print('Enter the net sales for') previous = float(input('- Prior menstruum:')) electric current = float(input('- Current period:')) # calculate the alter in percentage change = (current - previous) * 100 / previous # show the result if change > 0: result = f'Sales increase {abs(change)}%' else: result = f'Sales decrease {abs(modify)}%' impress(result) except ValueError: impress('Mistake! Please enter a number for internet sales.') except ZeroDivisionError: impress('Error! The prior cyberspace sales cannot be zero.')
Code language: Python ( python )
When you enter zip for the net sales of the prior period:
Code language: Shell Session ( shell )
Enter the net sales for - Prior period:0 - Current catamenia:120
… y'all'll get the post-obit error:
Code language: Shell Session ( vanquish )
Fault! The prior net sales cannot be zip.
It's a good practice to grab other general errors past placing the catch Exception
block at the stop of the listing:
try: # get input cyberspace sales print('Enter the internet sales for') previous = bladder(input('- Prior menstruation:')) electric current = float(input('- Current period:')) # calculate the change in pct change = (current - previous) * 100 / previous # show the result if modify > 0: result = f'Sales increase {abs(change)}%' else: consequence = f'Sales decrease {abs(alter)}%' print(consequence) except ValueError: print('Error! Delight enter a number for net sales.') except ZeroDivisionError: print('Mistake! The prior net sales cannot be null.') except Exception as mistake: print(fault)
Code language: Python ( python )
Summary
- Utilise Python
effort...except
argument to handle exceptions gracefully. - Use specific exception in the
except
block as much as possible. - Utilise the
except Exception
statement to grab other exceptions.
Did you discover this tutorial helpful ?
wilkersontrablinever.blogspot.com
Source: https://www.pythontutorial.net/python-basics/python-try-except/
Belum ada Komentar untuk "Python Try- Error Except Try Again"
Posting Komentar